How to Hide an Account Menu Item
Sometimes you may want to hide an item from the account menu. We have a filter to make this possible.
Add the following code to your theme’s functions.php
file.
/**
* Hide an account menu item.
*
* @param array $hidden
*
* @return array
*/
function iconic_hidden_pages( $hidden ) {
$hidden[] = 'example-page';
return $hidden;
}
add_filter( 'iconic_wap_hidden_pages', 'iconic_hidden_pages', 10, 1 );
The filter accepts 1 parameter:
$hidden
This is an array of page slugs. In this example we’re addingexample-page
as a hidden item.
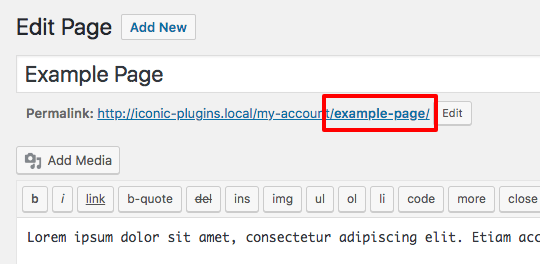
This page will now be hidden from the menu. You can add more pages by duplicating this line $hidden[] = 'example-page';
.
If you want to hide a menu item from a specific user role, you can do the following:
/**
* Hide an account menu item.
*
* @param array $hidden
*
* @return array
*/
function iconic_hidden_pages( $hidden ) {
if ( iconic_get_current_user_role() === 'wholesale' ) {
$hidden[] = 'example-page';
}
return $hidden;
}
add_filter( 'iconic_wap_hidden_pages', 'iconic_hidden_pages', 10, 1 );
/**
* Get current user role.
*
* @return bool|string
*/
function iconic_get_current_user_role() {
if( is_user_logged_in() ) {
$user = wp_get_current_user();
$role = ( array ) $user->roles;
return $role[0];
} else {
return false;
}
}
The code is much the same as before, except this time we’re using iconic_get_current_user_role()
to determine if the user has the wholesale
role. If they do, we’ll hide the example-page
menu item.
WooCommerce Account Pages
Add and manage pages in your WooCommerce “My Account” area using the native WordPress “Pages” functionality.
Was this helpful?
Please let us know if this article was useful. It is the best way to ensure our documentation is as helpful as possible.